Writing ALP application
How to write an application for ALP? Following the beginner samples from the
ALP package you can learn how to use the ASP pages to perform tasks and how to
combine the pages together to form an application. Let us examine this
application building process as if the application is something substantial that
would need all the major ALP application structural elements mentioned in the
"Configure ALP" chapter.
Creating the structure
On a WEB server (IIS for instance) creating a virtual site (virtual WEB
server) for each application can be expensive as effort and may even cost
additional expenses for DNS name, for an IP and so on. With ALP it is a matter
of a few mouse clicks in a folder and does not involve configuration of external
servers (such as DNS), nor adds any costs. Thus in ALP each application can
afford its own virtual ALP site. Only a reason related to the actual application
functionality makes it sensible to combine more than one applications in a
single Virtual ALP site if it would bring some benefits to the applications.
Thus the first step is to create a virtual ALP site. We can start by
creating a directory for our application - let it be c:\myapp.
Right click in the folder window to invoke the Windows Explorer's
context menu. There are 3 items added by the ALP Shell extensions. We need
the ALP Settings which will open the ALP Settings dialog for that folder.
In that dialog we can click Create new virtual ALP site in the current
directory:
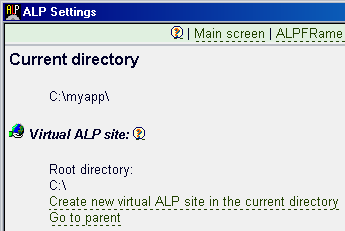
This will create a virtual ALP site and we will see that an alp.site
file appears in the folder:
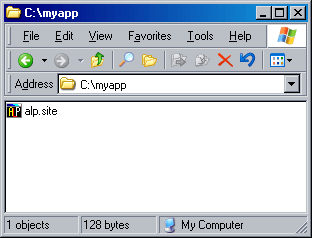
With this we have the basic structure, because the virtual ALP site
implicitly implies that an ALP Application begins in its root directory
and that application will use the ALP default settings which are good for
the most applications.
Now is time to start with the application, we may need to set
additional ALP settings for it, but it will become clear what we might
need as we progress, thus we will not add more settings for now.
A typical ALP application would need a global.asa file to
initialize certain application wide variables, set default values and so
on. For the sake of the example we will assume we are creating an
application that uses SQLite COM database. Suppose the application uses
single database and almost every page we are going to write will execute
some queries on it. Obviously we will make our work easier if we can open
this database once - when the application starts and use it from all the
pages without additional efforts. This means we will need the SQLite COM
object initialized in the global.asa. What else? We look in the
documentation to see what other objects we will need almost permanently.
One obvious candidate is the StringUtilities object which will help us
create the queries we will execute over the database. So let's compose a
global.asa that will do this for us:
<OBJECT RUNAT="SERVER"
ID="g_db"
PROGID="newObjects.sqlite.dbutf8"
SCOPE="Application"></OBJECT>
<OBJECT RUNAT="SERVER"
ID="g_su"
PROGID="newObjects.utilctls.StringUtilities"
SCOPE="Application"></OBJECT>
<SCRIPT RUNAT="SERVER" LANGUAGE="VBSCRIPT">
Sub Application_OnStart
g_db.Open Server.MapPath("/mydb.db")
g_db.AutoType = True
g_db.TypeInfoLevel = 4
End Sub
</SCRIPT>
We open the database file and we set general behavior parameters to the
database object. These parameters will cause it to convert the returned
results to their COM compatible types and report maximum type information
for each field returned by a query. We specify the path to the database
file using Server.MapPath to ensure it will always map in the root of our
virtual ALP site. Now we must save this as global.asa file and create the
database and design its structure using the SQLite Database Manager (we
will skip this step for brevity):
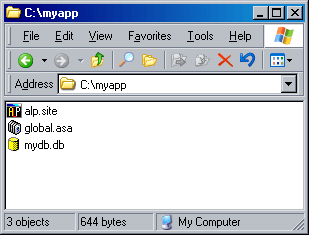
The global.asa file we just created will do a very important thing for
us - it will extend the ASP object model with the objects we defined
there. Note the ID attributes in bold - they define the variable names
under which we will be able to access the objects we created from any ASP
page in the application. The SCOPE attribute states that these objects
have application scope which means their lifetime will be the lifetime of
our application instance.
We are not going in this page to create a fully functional application,
but lets put some flesh over the application skeleton. Assume we have a
table named Clients in the database. Lets create a page that lists
them and put it into the application:
<html>
<head>
<title>Clients</title>
</head>
<body>
<table border="1">
<tr>
<th valign="top" nowrap>Name</th>
<th valign="top" nowrap>Email</th>
</tr>
<%
Set r = g_db.Execute("SELECT * FROM Clients ORDER BY Name")
For I = 1 To r.Count
%>
<tr>
<td valign="top"><%= r(I)("Name") %></td>
<td valign="top"><%= r(I)("Email") %></td>
</tr>
<%
Next
%>
</table>
</body>
</html>
Now we save this let say as list.asp. If we enter some data in
the table using the SQLite Database manager we can start the page to see
it working. We can use the ALP Shell extensions again:
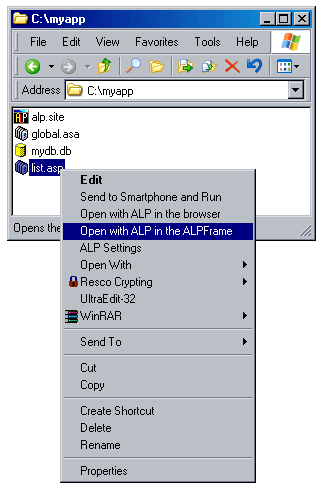
Right click the saved file and choose Open with ALP in ALPFrame.
The page will show something like this (depending on what has been entered
into the table):
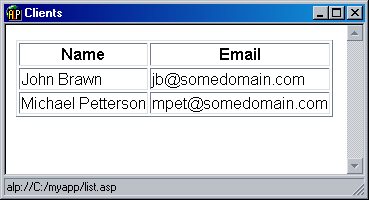
Notice how the page uses the database without initializing the object -
it is already opened in the global.asa file. In such an application we
would need pages that will add/change the database. We will limit
ourselves in this example to a very simple insert page:
<html>
<head>
<title>Insert client</title>
</head>
<body>
<FORM METHOD="POST" ACTION="<%= Request.ServerVariables("SCRIPT_NAME") %>">
Name <input NAME="Name" type="text" size="20"><br>
Email <input NAME="Email" type="text" size="20"><br>
<input type="SUBMIT" value="Insert">
</FORM>
<%
If Request.ServerVariables("REQUEST_METHOD") = "POST" Then
qry = g_su.Sprintf("INSERT INTO Clients (Name,Email) VALUES (%q,%q)", _
Request("Name"),Request("Email"))
g_db.Execute qry
End If
%>
<A HREF="list.asp">To the list</A>
</body>
</html>
We can save it as insert.asp and run it to add some new entries
and go the the list.asp page to see them. As in the other page we do
not need to use Server.CreateObject yet - the most needed objects are
already created in the global.asa file.
Continuing with the application we will certainly need more objects and
not all of them will be common for the entire application. Therefore in
some pages we will use Server.CreateObject to create them only where they
are needed. We may find out that some more objects can be placed in our
global.asa, that we may need some application specific settings and so on.
Of course this will depend on what exactly we are going to do. We will
complete this page by adding a default.htm page which will provide the
user with links to the pages we created above and serves as application
entrance. This is a good opportunity to demonstrate how the unattended
execution protection can be turned on. Our default.htm page is obviously
harmless - it is a HTML page and cannot do any harm to the system, but we
have that insert.asp page that adds data to the database and
perhaps in the process we will add more such pages and some of them may
perform even more critical operations (for example import/export files).
This makes it sensible to turn on the unattended execution protection. To
do so we go again to the ALP Settings shell extension (see the beginning),
but we click on Set specific settings for this directory this time.
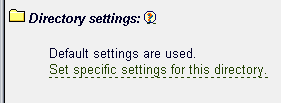
In the following dialog we check the Prevent unattended execution
checkbox and we update the settings.
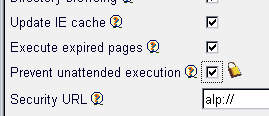
Finally our directory looks now like this:
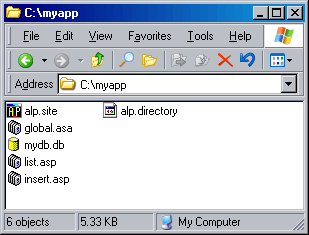
Now when the application is entered by double clicking the alp.site
file or from an especially created shortcut pointing to it, or by an URL
pointing the directory (alp://c:/myapp) or the default.htm (alp://c:/myapp/default.htm)
everything will be ok. But if by any chance the application is started by
an URL that points another file (for example - alp://c:/myapp/list.asp
or alp://c:/myapp/insert.asp) ALP will show an error message like
this one:
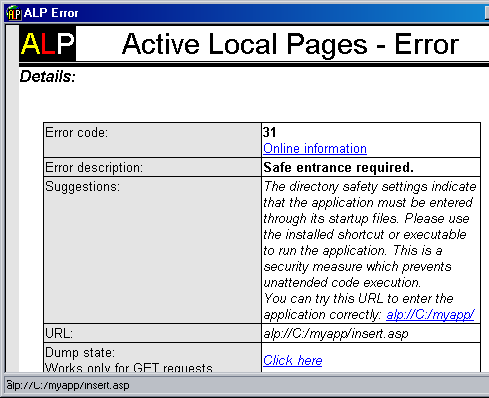
Thus ALP will forbid the execution of these pages unless the
application is entered through a default document. The default documents
are configured in the same dialog where is the Prevent unattended
execution option. We can configure single such file or more than one,
in any case we must ensure none of them performs potentially harmful
operations. This simple mechanism ensures that the user has actually
entered the application and that a page from the application is not
invoked by a trap put by a malicious user (for example link in an online
page). If the application is highly sensitive the developer can go further
and use more of the ALP protection features.
Progressing with the application we may need to set some specific
settings for the ASP processor - to do so we enter the ALP Settings as
before and go to the Application settings area, then we edit the .asp and
may be also the.asa file extension settings if the feature is needed in
the both regular ASP pages and the global.asa. If this is an application
that will be distributed and we have a developer license we enter the
virtual ALP site settings dialog again and import the license from the
template alp.site file received after the purchase.
|